CSRF Attack and CSRF Tokens
Welcome to Secumantra! In this post we will understand Cross-Site Request Forgery attack, commonly known as CSRF or XSRF attack. It is one of the common attacks observed for web applications and has been there in OWASP Top Ten for many years.
Introduction and OWASP Overview
In a CSRF attack an end user’s browser is tricked by an attacker into executing a malicious web request using victim’s authenticated credentials. It happens in the background and end user doesn’t even know about it.
Successful CSRF attacks can have serious consequences. This may cause actions to be performed on the website that can include inadvertent client or server data leakage, change of session state, or manipulation of an end user’s account. So let’s see how CSRF attack works and how you can prevent it.
To start with, let’s talk briefly about different OWASP parameters related to CSRF attack.
- Threat Agents can be anyone who can trick your users into submitting a request to your website. Any website or other HTML feed that your users access could do this
- Exploitability is average where an attacker creates a forged HTTP request and tricks a victim into submitting them via image tags, XSS or some other techniques. If the user is authenticated, the attack succeeds.
- The prevalence of CSRF is very wide spread, but the detectability is also very easy. CSRF takes advantage of web applications that allow attackers to predict all the details of a particular action. Since browsers send credentials like session cookies automatically, attackers can create malicious web pages which generate forged requests that are indistinguishable from legitimate ones. Fortunately detection of CSRF flaw is fairly easy via penetration testing or code analysis.
- Technical impact of the CSRF attack is moderate in general and it really depends on the nature of the vulnerable application. Attackers can cause victims to change any data the victim is allowed to change or perform any function the victim is authorized to use.
- Business impact of CSRF attack depends on nature of the business and nature of the data. It depends on the individual site and exploiting it can do considerable damage to not only yo the customers but also to company’s reputation.
Example flow and Consequences
Let’s imagine a simple workflow to understand how CSRF attack happens –
- Use is logged in to a legitimate web application, let’s say example.com. Websites use cookies to identify a user, or to retain a user’s logged in session on the website. Session cookies typically contain a unique ID, which is an identifier the web application uses to identify a particular logged in user. Therefore, when a cookie is set for a specific website, the web browser sends it along with every HTTP request it issues to that website to retain the logged in session.
- Now user visits another website, consider as an attacker’s domain (hack-web-app.com). It can also come by another way like some social media or clicking on a Facebook link.
- When attacker’s domain is visited by the user, the attacker tricks the user’s browser to into sending a request that changes the user’s data in the application hosted on example.com
- When request is sent to domain example.com, user’s authentication cookie is appended as usual to outgoing cross site request. Once this malicious request is processed by the web application user’s data is changed which was not initiated by the user himself. It can be further extended to change user credentials as well.
This is called the cross-site request forgery aka CSRF attack! This is a totally independent third party site that could trick the browser into updating the user data on our legitimate site.
Let’s imagine attacker tricks the user’s browser into sending a login request with attacker’s credentials. Once the request is processed, user is switched to the attacker’s account. If the user didn’t notice that he has been switched to the attackers account, then the credit card data user will enter will be entered actually to attacker’s account.
How CSRF Attack Works?
Now the question is how attacker can trick user’s browser into sending a request into another domain? What makes a CSRF attack possible?
- Authenticated sessions are persisted via cookies. The cookie is sent with every request to the domain.
- The attacking site reconstructs a legitimately formed request to the target site, but with malicious payload.
- The victim’s browser is tricked into issuing the request. The target website views it as a legitimate request looking at the structure and cookie.
Here is a very basic example showing Javascript code, hosted on attacker’s domain –
<html>
<body>
Hello!
<script>
var xhr = new XMLHttpRequest();
xhr.open("POST", "https://example.com/profile.php", true);
xhr.SetRequestHeader("Content-Type", "application/x-www-form-urlencoded");
xhr.withCredentials = true;
var body = "[email protected]&action=Change+email";
xhr.send(body);
</script>
</body>
</html>
We can see that a POST request is reconstructed and sent using the parameters required for example.com. It is a forged request and not a legitimate one. Once the request is executed, the mail is changed into [email protected]. All this happens silently in the background which is again a great thing from attacker’s point of view.
Now this can be further extended by the attacker to change the user’s password. It is just a matter of constructing a post request and send it using authentication cookies from example.com. This way complete account can also be taken over.
Anti-Forgery Token (Anti-CSRF Token)
Let’s try to figure out the reason and how we can fix this problem.
We observed that CSRF attack works because they are predictable. In other words, attacker is just creating a legitimate looking request (with required parameters and cookie) with a malicious payload.
So we can mitigate this risk if we could add some randomness to the process. This is done via implementing an Anti-CSRF token. It is a type of server side CSRF protection. This token is a piece of data (random string) known only to the user’s browser (inside a session variable) and the legitimate web application.
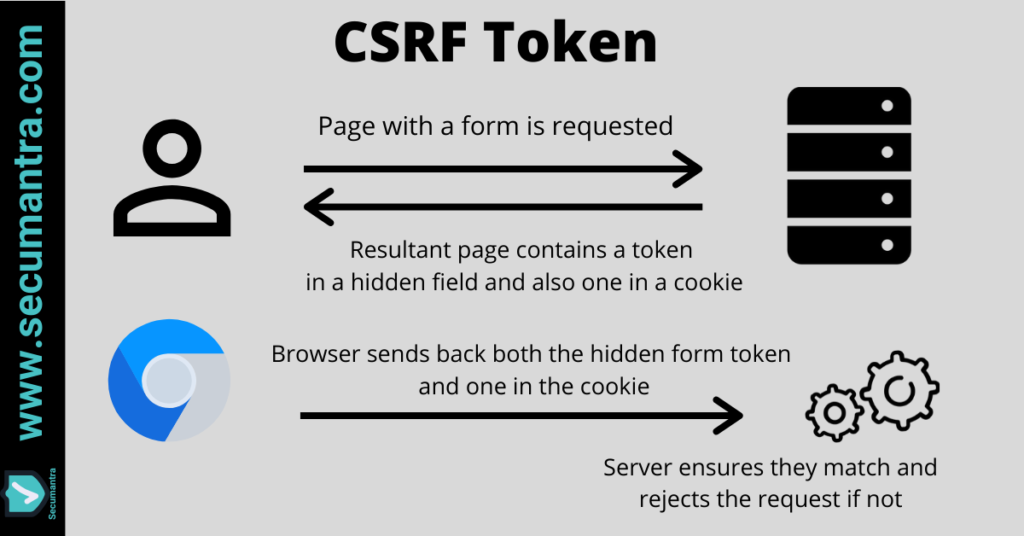
- Let’s imagine a request and a response sequence to understand how CSRF token works. So user requests a page from a server and when the server responds it has a token in a hidden field in the form of the page and it also has a token in the response header via a cookie.
- Now when the form is submitted, token in the hidden form field and token in the form cookie is also sent along. We know that any cookie set against the domain name is automatically sent, but now the hidden form field is also sent. This is the randomness because attacking site doesn’t know what value token should be in the hidden form field.
- When a request with Anti-CSRF token is received by the web application, it first verifies the CSRF token. If it is correct, then only request is processed by the web application.
Note that Attacker doesn’t know the Anti-CSRF token for the user, so attacker cannot reconstruct the legitimate the request that could change user’s data in the web application hosted on another domain. This way CSRF attack is prevented.
This is a very effective way to mitigate the risk against CSRF attack. In fact it is really the only major defense we have against CSRF.
CSRF tokens should be treated as secrets and handled in a secure manner throughout their lifecycle. An approach that is normally effective is to transmit the token to the client within a hidden field of an HTML form that is submitted using the POST method.
Apart from implementing CSRF tokens, the server application must verify that each sensitive HTTP request contains the correct CSRF token. Token string must be long enough and unpredictable like GUIDs.
Browser Defense
CSRF risk may be exploited by using cross-origin resource sharing (CORS). Different browsers provide varying levels of defense against unintended CORS requests and prohibit one domain maliciously interacting with another domain.
Although modern web browsers prevent CSRF attacks due to same-origin policy restrictions, we should never rely on the native browser defenses. It always better to make sure that you have implemented robust security measures on the individual website. So always write secure code which for CSRF attack ultimately means Anti-CSRF tokens.
Summary
CSRF attack happens when a legitimate request is reconstructed into one with a malicious intent. The authenticated state of the victim is abused and the browser is tricked (using social engineering) into issuing the request. A successful CSRF attack can perform actions like transferring funds, changing user’s credentials or can even compromise the entire web application. The only real mitigation is to use anti-forgery tokens. Although modern browsers offer some native defenses but we should not rely on them entirely.
As awareness of CSRF has increased and web applications are implementing CSRF protection techniques (the most common being anti-CSRF tokens), there has been significant reduction in overall CSRF risk. Overall ranking of CSRF attack has also went down in OWASP Top 10 list in recent years.
Thank you for reading. Happy Secure-Coding!